Generate Test Suites As You Code
Stop writing tests manually. DebuggAI watches your app as you develop and automatically builds comprehensive test suites that catch bugs before they reach production.
Effortless Test Generation
DebuggAI monitors your application as you develop, learning from real usage patterns to automatically generate comprehensive test suites.
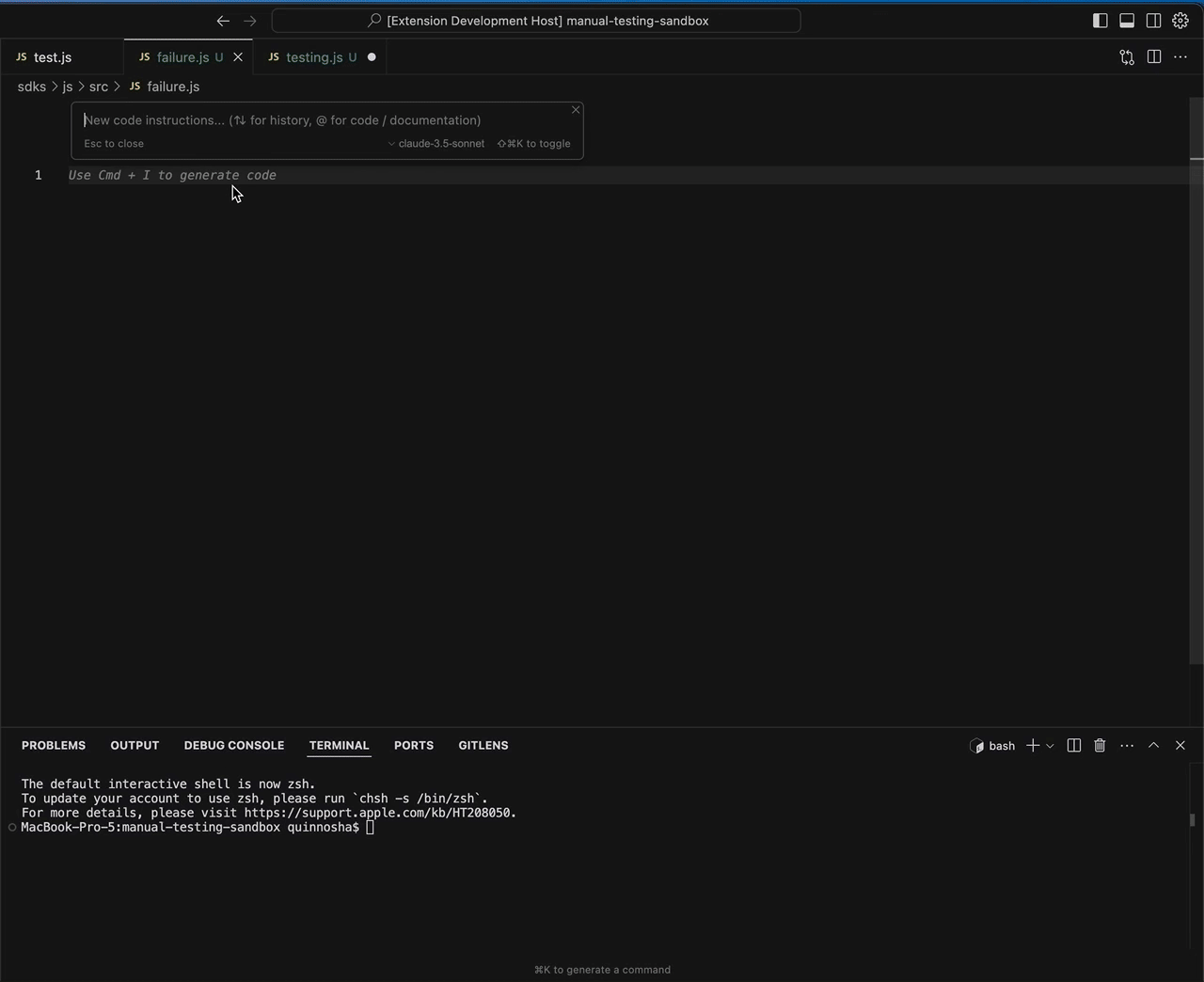
1. Run Your App
Simply run your application locally with our lightweight SDK. It works with any framework or language.
2. Automatic Monitoring
Our SDK silently monitors function calls, edge cases, and error states as you interact with your app.
3. AI Test Generation
DebuggAI analyzes the collected data and generates comprehensive test suites tailored to your codebase.
4. Seamless Integration
Tests are automatically added to your project, ready to run in your existing test framework.
Comprehensive Test Coverage
DebuggAI doesn't just generate basic tests. It creates comprehensive test suites that cover edge cases, error handling, and performance scenarios.
Comprehensive Test Coverage
Automatically generate tests for edge cases, error handling, and happy paths based on real usage patterns.
Framework Agnostic
Works with any testing framework including Jest, Mocha, Pytest, JUnit, and more.
CI/CD Integration
Seamlessly integrates with your existing CI/CD pipeline to run generated tests automatically.
Real-time Test Generation
Tests are generated in real-time as you develop, ensuring your test suite grows with your codebase.
Regression Prevention
Automatically creates tests for fixed bugs to prevent regressions and ensure issues stay resolved.
Performance Testing
Identifies performance bottlenecks and generates tests to ensure your code meets performance requirements.
AI-Generated Test Examples
See how DebuggAI generates comprehensive tests for different languages and frameworks.
import { expect } from 'chai';
import sinon from 'sinon';
import { AuthService } from '../services/AuthService';
import { UserRepository } from '../repositories/UserRepository';
describe('AuthService', () => {
let authService;
let userRepository;
beforeEach(() => {
userRepository = new UserRepository();
authService = new AuthService(userRepository);
});
describe('login', () => {
it('should return a token when credentials are valid', async () => {
// Arrange
const validCredentials = {
username: 'testuser',
password: 'password123'
};
const mockUser = {
id: 1,
username: 'testuser',
passwordHash: '$2a$10$X7UrY9Hx1PBxVV6X4Ygk2uQ8z8.9QVJ5Z.3XmP9Qf4pX5ZzLa',
roles: ['user']
};
sinon.stub(userRepository, 'findByUsername').resolves(mockUser);
sinon.stub(authService, 'verifyPassword').returns(true);
sinon.stub(authService, 'generateToken').returns('valid-jwt-token');
// Act
const result = await authService.login(validCredentials);
// Assert
expect(result).to.have.property('token', 'valid-jwt-token');
expect(result).to.have.property('user');
expect(result.user).to.have.property('id', 1);
expect(result.user).to.have.property('username', 'testuser');
expect(result.user).to.not.have.property('passwordHash');
});
it('should throw an error when username is not found', async () => {
// Arrange
const invalidCredentials = {
username: 'nonexistentuser',
password: 'password123'
};
sinon.stub(userRepository, 'findByUsername').resolves(null);
// Act & Assert
try {
await authService.login(invalidCredentials);
expect.fail('Expected error was not thrown');
} catch (error) {
expect(error.message).to.equal('Invalid username or password');
expect(error.statusCode).to.equal(401);
}
});
it('should throw an error when password is incorrect', async () => {
// Arrange
const invalidCredentials = {
username: 'testuser',
password: 'wrongpassword'
};
const mockUser = {
id: 1,
username: 'testuser',
passwordHash: '$2a$10$X7UrY9Hx1PBxVV6X4Ygk2uQ8z8.9QVJ5Z.3XmP9Qf4pX5ZzLa',
roles: ['user']
};
sinon.stub(userRepository, 'findByUsername').resolves(mockUser);
sinon.stub(authService, 'verifyPassword').returns(false);
// Act & Assert
try {
await authService.login(invalidCredentials);
expect.fail('Expected error was not thrown');
} catch (error) {
expect(error.message).to.equal('Invalid username or password');
expect(error.statusCode).to.equal(401);
}
});
// Additional tests generated based on observed edge cases
it('should handle null credentials gracefully', async () => {
try {
await authService.login(null);
expect.fail('Expected error was not thrown');
} catch (error) {
expect(error.message).to.equal('Credentials are required');
}
});
});
});
Why Use AI-Generated Tests?
DebuggAI's test generation capabilities deliver significant advantages for development teams of all sizes.
Save Development Time
Reduce time spent writing and maintaining tests by up to 80%, allowing your team to focus on building features.
Increase Code Quality
Comprehensive test coverage leads to more robust code with fewer bugs and regressions.
Catch Bugs Earlier
Identify and fix issues during development before they reach production or affect users.
Improve Developer Experience
Eliminate the tedious aspects of testing while maintaining high-quality standards.
Learn Best Practices
AI-generated tests follow industry best practices, helping your team learn and improve their testing skills.
Streamline CI/CD
Integrate with your existing CI/CD pipeline for seamless test execution and deployment.
Frequently Asked Questions
Find answers to common questions about our AI test generation capabilities.
Stop Writing Tests. Start Building Features.
Join forward-thinking development teams who have reduced testing time by up to 80% while improving code quality with DebuggAI's automatic test generation.
Get started today. No credit card required.